Programming has three key elements: sequence, selection, and iteration. These are the basics that every programmer needs to know. They help create efficient and well-structured code. Whether you’re new to coding or have been doing it for years, knowing these basics is key.
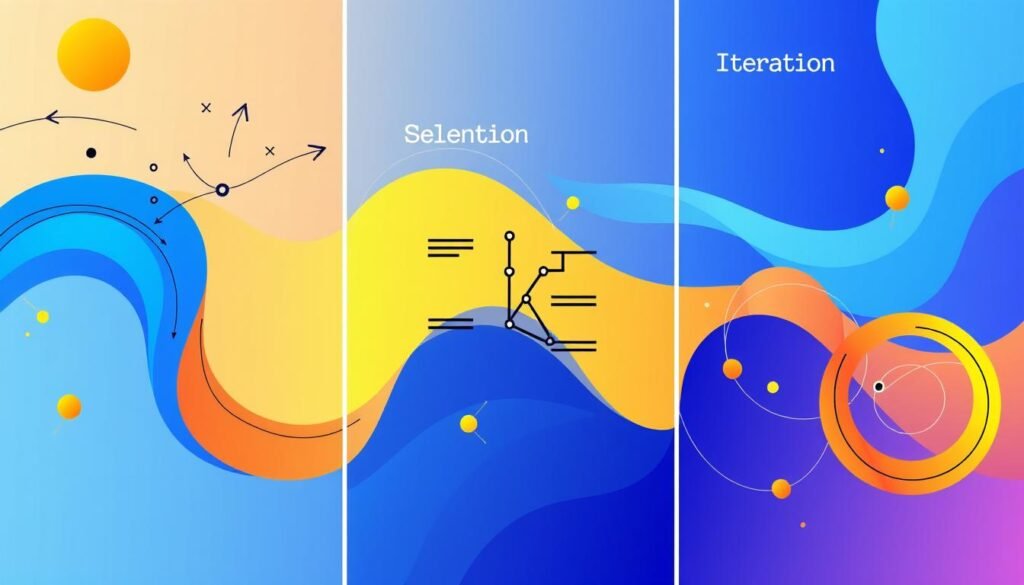
Key Takeaways
- Programming constructs are the fundamental building blocks of all code, including sequence, selection, and iteration.
- Mastering these constructs is essential for writing efficient, structured, and versatile software programs.
- Sequence, selection, and iteration are used in all programming languages, from beginner-level to advanced.
- Understanding the role and implementation of these constructs is crucial for success in computer science and software development.
- Applying these constructs correctly can greatly improve the readability, maintainability, and performance of your code.
Understanding Programming Fundamentals: A Beginner’s Guide
Programming is all about the basics. These basics, or programming constructs, are the foundation of all software. For beginners, learning these programming concepts is key to becoming a skilled developer.
Why Programming Constructs Matter
Programming constructs like sequential execution, decision-making, and looping are the code’s building blocks. Mastering these code structure principles helps developers make efficient, maintainable software. These concepts are universal, useful across many programming languages.
Core Building Blocks of Code
The three main programming constructs are:
- Sequence: Instructions are executed one after another.
- Selection: Code makes choices based on conditions.
- Iteration: Loops repeat a set of instructions.
Knowing these constructs helps developers create complex software solutions. Whether you’re new or experienced, reviewing these basics can improve your skills in programming concepts, code structure, and software engineering principles.
“The foundation of any great software is built upon a deep understanding of the fundamental programming constructs.”
The Three Basic Programming Constructs
In programming, three key elements are crucial: sequence, selection, and iteration. These are the core parts that help programmers make powerful apps.
The sequence construct is the simplest. It makes sure code runs in order from top to bottom. This is the base for more complex coding.
The selection construct lets programs make choices. With if-else or switch-case statements, code can change based on conditions. This makes programs more flexible.
The iteration construct, or loops, repeats tasks until a condition is met. This is key for automating tasks and handling big data. It also makes apps more interactive.
Understanding sequence, selection, and iteration is essential. They are the foundation of programming. With these basics, programmers can make complex, efficient, and user-friendly apps.

Construct | Definition | Examples |
---|---|---|
Sequence | Linear execution of instructions | Executing lines of code in the order they appear Performing tasks sequentially |
Selection | Decision-making based on conditions | If-else statements Switch-case statements |
Iteration | Repetition of a set of instructions | For loops While loops Do-while loops |
“The three basic programming constructs are the fundamental building blocks that enable programmers to create sophisticated, dynamic, and efficient applications.”
Sequential Programming: The Foundation of Code Execution
At the heart of every computer program is a key principle: linear execution. Code is run step-by-step, line by line. This precise code order shapes the program’s actions. Understanding this step-by-step programming is crucial for any coder.
Line-by-Line Execution
In sequential programming, each instruction is followed by the next. Each line of code is executed in its given order. This ensures the program works as planned, in a controlled way. Developers must think about the code order carefully, as small changes can greatly affect the outcome.
Implementation Examples in Different Languages
Sequential programming is not limited to one language. It applies to all, from Python to C++. Each language has its own way of handling linear execution. Looking at examples in different languages can help you grasp this key concept better.
Best Practices for Sequential Programming
To write good sequential code, follow some key practices. Keep your code clear and well-commented. Break down big tasks into smaller, easier steps. Also, test and debug your program often. By doing these things, you can make step-by-step programming that works well and is easy to keep up with.
“The best way to learn programming is to write programs, because that’s how you learn the language, the concepts, and the process of creating software.”
– Brian Kernighan, co-author of “The C Programming Language”
Selection Statements: Making Decisions in Code
In programming, making decisions is key. *Conditional statements*, like if-else and switch-case, help code branch and respond to various situations. These *branching* tools let programs run different instructions based on certain conditions. This helps developers make software that can change and adapt.
The if-else statement is a basic tool for making decisions in code. It checks a condition and runs one code block if it’s true, and another if it’s false. This simple tool is the base of conditional statements and lets programmers handle complex choices.
The switch-case statement is another common choice. It checks an expression and runs a specific code block based on its value. The switch-case is great for handling many possible outcomes, making code more concise and easy to read.
Learning these *conditional statements* and *branching* methods is essential for new programmers. By knowing how to make smart choices in code, developers can build flexible and smart apps. These apps can handle many scenarios and user inputs.
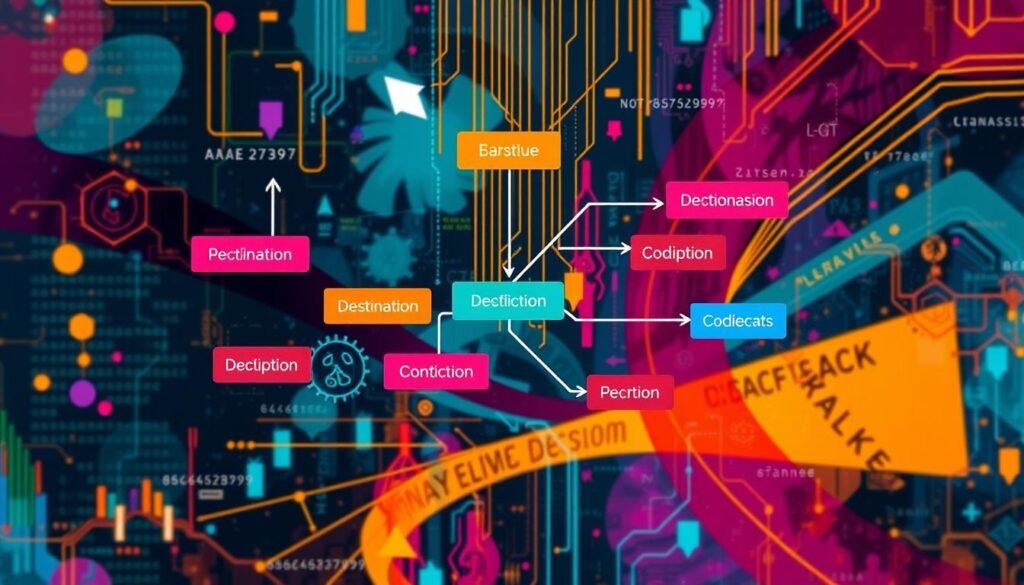
“Conditional statements are the heartbeat of any programming language, allowing code to make decisions and adapt to different situations.”
Iteration and Loops: Repeating Code Efficiently
In programming, loops are key to making code run smoothly. They let developers repeat code blocks, making programming easier and more efficient. Let’s explore the various loop types and when to use them to meet your coding needs.
Different Types of Loops
Programmers have many loop types to choose from, each for a different purpose:
- for loops – Great for known number of iterations.
- while loops – Best for unknown iterations, continuing as long as a condition is true.
- do-while loops – Similar to while loops but runs at least once, regardless of the condition.
When to Use Each Loop Type
The right loop type depends on your programming task’s needs:
- Choose for loops for known iterations or when working with collections like arrays or lists.
- Go with while loops for unknown iterations or when a condition needs to be met.
- Use do-while loops to ensure code runs at least once, even if the condition is false initially.
Common Loop Patterns
Developers often use known loop patterns to tackle common challenges. Some top patterns include:
- Counting loops – A for loop for a set number of iterations.
- Sentinel-controlled loops – A while loop that runs until a certain condition is met.
- Nested loops – One loop inside another, useful for handling complex data structures.
Knowing the different loop types and their uses helps programmers create better, more efficient code. This improves the quality of software solutions.
Real-World Applications and Examples
The three basic programming constructs – sequence, selection, and iteration – are key in many areas. They help create everything from simple scripts to complex software. These constructs make modern technology work better and faster.
Mobile apps are a great example of how these constructs are used. Developers use sequence to make sure things happen in order. They use selection statements for different user inputs and loops for tasks that need to be done over and over. This makes apps work smoothly and efficiently.
In web development, these constructs are just as important. They help structure web pages and make content dynamic. Loops are used for tasks like creating menus or filling data sections. This shows how widely used these concepts are in software development.
FAQ
What are the three basic programming constructs?
The three basic programming constructs are sequence, selection, and iteration. These are key for writing efficient code. They are the foundation of all programming languages and software development.
Why are programming constructs important?
Programming constructs are key because they help create organized, efficient code. They are the basis for all programming languages. This lets developers build complex software systems.
How does sequential programming work?
Sequential programming runs code line by line, in the order written. It’s the basic way code is executed. Knowing how to write clear, efficient sequential code is important.
What are the different types of selection statements?
Selection statements, like if-else and switch-case, let programs make decisions based on conditions. These statements are vital for adding decision-making logic to code.
How do loops and iteration work in programming?
Loops and iteration allow code to repeat efficiently. There are different loops, like for loops and while loops, each for specific needs. Choosing the right loop is important for programming.
Can you provide real-world examples of the three basic programming constructs?
The three basic programming constructs are used in many software applications. They are crucial for both simple scripts and complex systems. These concepts are essential for creating functional and efficient programs.